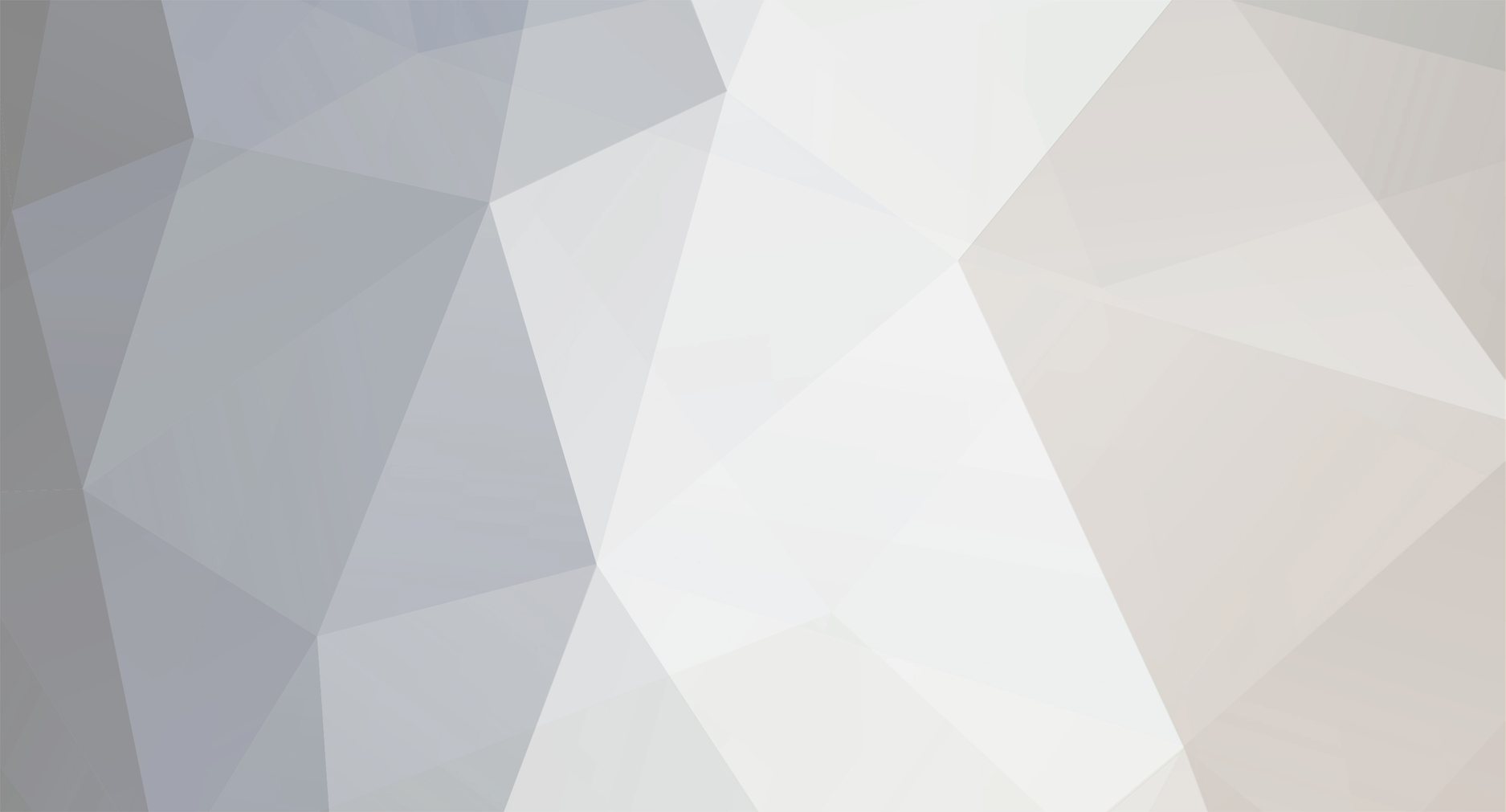
Glenn9999
Platinum SponsorContent Type
Profiles
Forums
Events
Everything posted by Glenn9999
-
Listing Patch History
Glenn9999 posted a topic in Programming (C++, Delphi, VB/VBS, CMD/batch, etc.)
As someone may or may not know, you can look in the registry at HKLM\SOFTWARE\Microsoft\Updates and find a list of patches by KB number and file names spread among all the subkeys for some things for that main link. So, I thought it might be interesting to try and aggregate the information. You can see an image of the final thing I came up with below: I say "patch history on Windows XP" because I can't really say this works on anything else at the moment (haven't tested it, so I don't know, but you can always try it). As for Windows 95/98/ME, I could probably make it work there, but I can't see on my copy of Windows ME that there's much there to look at. So I stuck a check for an NT based operating system on this. I spot checked a few of the entries and all seems well. Hopefully this might be useful to someone. Highlights from the code for a Delphi programming example standpoint: 1) A rudimentary use of TTreeView. 2) Demonstrates recursion through the registry. 3) Demonstrates the use of TList and sorting. unit updunit; interface uses Windows, Messages, SysUtils, Classes, Graphics, Controls, Forms, Dialogs, ComCtrls, StdCtrls, registry, ExtCtrls; type TForm1 = class(TForm) TreeView1: TTreeView; UpdBtn: TButton; SaveBtn: TButton; SaveDialog1: TSaveDialog; RadioGroup1: TRadioGroup; procedure UpdBtnClick(Sender: TObject); procedure SaveBtnClick(Sender: TObject); procedure FormCreate(Sender: TObject); private { Private declarations } public { Public declarations } end; type file_record = record package_name: string; filename: string; end; pfile_record = ^file_record; var Form1: TForm1; file_value: TList; file_list: pfile_record; implementation {$R *.DFM} function os_is_nt: Boolean; { returns whether the OS is NT based or not } var osvinfo: TOsVersionInfo; begin { get windows version } osvinfo.dwOSVersionInfoSize := Sizeof(osvinfo); GetVersionEx(osvinfo); os_is_nt := (osvinfo.dwPlatformId = VER_PLATFORM_WIN32_NT); end; function get_pn(keyname: string): string; { parse out name of patch from registry entry } var filelist_pos: integer; i: integer; begin filelist_pos := pos('\Filelist', keyname); i := filelist_pos - 1; while keyname[i] <> '\' do dec(i); get_pn := copy(keyname, i+1, filelist_pos - i - 1); end; procedure recurse_list(keyname: string); { recurse through patch list, identify listed files } var appcheck: TRegistry; mainkeys: TStringList; mainvalues: TStringList; count1, count2: integer; begin appcheck := TRegistry.Create; appcheck.rootkey := HKEY_LOCAL_MACHINE; if appcheck.openkey(keyname, false) then begin if pos('Filelist', keyname) > 0 then begin mainvalues := TStringList.Create; appcheck.getvaluenames(mainvalues); if mainvalues.count > 0 then begin New(file_list); file_list^.package_name := get_pn(keyname); for count2 := 0 to mainvalues.count - 1 do if mainvalues.strings[count2] = 'FileName' then file_list^.filename := UpperCase(appcheck.ReadString('FileName')); file_value.add(file_list); end; mainvalues.Destroy; end; mainkeys := TStringList.Create; appcheck.getkeynames(mainkeys); for count1 := 0 to mainkeys.count - 1 do recurse_list(keyname + '\' + mainkeys.strings[count1]); mainkeys.Destroy; end; appcheck.Destroy; end; function filename_sort(Item1, Item2: Pointer): integer; { -1 if Item 1 < Item 2 0 if Item 1 = Item 2 1 if Item 1 > Item 2 } var PItem1: pfile_record; PItem2: pfile_record; begin Result := 0; PItem1 := Item1; PItem2 := Item2; if PItem1^.Filename < PItem2^.Filename then begin result := -1; exit; end; if PItem1^.Filename > PItem2^.Filename then begin result := +1; exit; end; if PItem1^.Filename = PItem2^.Filename then begin if PItem1^.package_name < PItem2^.package_name then begin result := -1; exit; end; if PItem1^.package_name > Pitem2^.package_name then begin result := +1; exit; end; if PItem1^.package_name = Pitem2^.package_name then begin result := 0; exit; end; end; end; function packagename_sort(Item1, Item2: Pointer): integer; { -1 if Item 1 < Item 2 0 if Item 1 = Item 2 1 if Item 1 > Item 2 } var PItem1: pfile_record; PItem2: pfile_record; begin Result := 0; PItem1 := Item1; PItem2 := Item2; if PItem1^.package_name < PItem2^.package_name then begin result := -1; exit; end; if PItem1^.package_name > PItem2^.package_name then begin result := +1; exit; end; if PItem1^.package_name = PItem2^.package_name then begin if PItem1^.filename < PItem2^.Filename then begin result := -1; exit; end; if PItem1^.Filename > Pitem2^.Filename then begin result := +1; exit; end; if PItem1^.Filename = Pitem2^.Filename then begin result := 0; exit; end; end; end; procedure TForm1.UpdBtnClick(Sender: TObject); const updkey: string = 'SOFTWARE\Microsoft\Updates'; var tnode: TTreeNode; oldpname: string; cbvar: string; i: integer; begin UpdBtn.Enabled := false; SaveBtn.Enabled := false; RadioGroup1.Enabled := false; TreeView1.Items.BeginUpdate; TreeView1.Items.Clear; file_value := TList.Create; recurse_list(updkey); case RadioGroup1.ItemIndex of 0: file_value.sort(packagename_sort); 1: file_value.sort(filename_sort); end; i := 0; file_list := file_value[i]; repeat OldPName := ''; if RadioGroup1.ItemIndex = 0 then begin cbvar := file_list^.package_name; TNode := TreeView1.Items.Add(nil, cbvar); repeat if file_list^.filename <> OldPName then begin TreeView1.Items.AddChild(TNode, file_list^.filename); OldPName := file_list^.filename; end; inc(i); Dispose(file_list); file_list := file_value[i]; until (cbvar <> file_list^.package_name) or (i = file_value.count - 1); end; if RadioGroup1.ItemIndex = 1 then begin cbvar := file_list^.filename; TNode := TreeView1.Items.Add(nil, cbvar); repeat if file_list^.package_name <> OldPName then begin TreeView1.Items.AddChild(TNode, file_list^.package_name); OldPName := file_list^.package_name; end; inc(i); Dispose(file_list); file_list := file_value[i]; until (cbvar <> file_list^.filename) or (i = file_value.count - 1); end; until (i = file_value.count - 1); { travel all, count nodes on main parents } for i := 0 to (TreeView1.Items.Count-1) do begin If TreeView1.Items[i].Parent = nil then TreeView1.Items[i].Text := TreeView1.Items[i].Text + ' (' + IntToStr(TreeView1.Items[i].Count) + ')'; end; TreeView1.Items.EndUpdate; { dispose memory here } file_value.Free; UpdBtn.Enabled := true; SaveBtn.Enabled := true; RadioGroup1.Enabled := true; end; procedure TForm1.SaveBtnClick(Sender: TObject); begin if SaveDialog1.Execute then if FileExists(SaveDialog1.Filename) then begin if MessageDlg('Overwrite this file?', mtWarning, [mbYes, mbNo], 0) = mrYes then TreeView1.SaveToFile(SaveDialog1.FileName); end else TreeView1.SaveToFile(SaveDialog1.FileName); end; procedure TForm1.FormCreate(Sender: TObject); begin if not os_is_nt then begin MessageDlg('Your OS is not supported.', mtWarning, [mbOK], 0); halt(1); end; end; end. You can download the project (Turbo Delphi 2006) here: http://rsgp0g.bay.livefilestore.com/y1p-Nx...CK.ZIP?download -
Using System Restore
Glenn9999 replied to Glenn9999's topic in Programming (C++, Delphi, VB/VBS, CMD/batch, etc.)
I redid the code a bit to show what is going on a little more at each step, and recompiled it within Turbo Delphi 2006. Files in the link below. http://rsgp0g.bay.livefilestore.com/y1pDdy...ls.zip?download -
This is intended to describe how to use the System Restore facility that exists in Windows XP in your Delphi program. Of course, this can be useful to find out how to use it for other languages. System restore enables the user to revert the computer to a previous state before that change is made. You will see it done commonly with install programs, but can also be done with any kind of code or registry change made to a system. Sample code, including a unit and a main program will be shown. Using System Restore requires that the SRCLIENT.DLL be present on the system. The general recommendation is to load the functions dynamically, so you will see that in the initialization of the unit. There are two functions that interest us: 1) 'SRSetRestorePointW' (also an A version for ANSI, W is Unicode) 2) 'SRRemoveRestorePoint' The first does everything we want in the creation of a restore point. The second removes restore points. In the creation of a restore point, a call is made to start the restore point, then the program does what is restorable, then a call to end the point is made. There is also the option to cancel a restore point. Calls for all three options exist in the unit below, along with the remove restore point call. Specific code examples can be gleaned from it. Also, a error string routine is provided for documentation purposes. When a restore point is made, an index number is created. This index number is provided in the function call of the unit. This number is required to make any subsequent references to the restore point. Sample code is provided below to handle the unit calls. Button1 creates a restore point session where a test file is written. Button2 will delete all system restore points. To test the restore point function (Button1): 1) Run the program and use the Button1 option. You will see a text file named TEST.DLL file created. 2) Go into the System Restore Utility, select to restore using the point name created. 3) once the reboot is completed, you should not find the TEST.DLL file anymore. Unit Code: unit sysrestore; { system restore access unit - provides access to the system restore function of Windows in order to set/cancel a restore point. Created using Turbo Delphi 2006 by Glenn9999 at msfn.org } interface uses windows; const { restore point types } APPLICATION_INSTALL = 0; APPLICATION_UNINSTALL = 1; DEVICE_DRIVER_INSTALL = 10; MODIFY_SETTINGS = 12; CANCELLED_OPERATION = 13; { event types } BEGIN_SYSTEM_CHANGE = 100; END_SYSTEM_CHANGE = 101; { other stuff } MAX_DESC = 256; type int64 = comp; { comment this if you are on a Delphi that supports int64 } restoreptinfo = packed record dwEventType: DWord; dwRestorePtType: DWord; llSequenceNumber: int64; szDescription: array[0..max_desc] of widechar; end; prestoreptinfo = ^restoreptinfo; statemgrstatus = packed record nStatus: DWord; llSequenceNumber: int64; end; pstatemgrstatus = ^statemgrstatus; set_func = function(restptinfo: prestoreptinfo; status: pstatemgrstatus): boolean; stdcall; remove_func = function(dwRPNum: DWord): DWord; stdcall; var DLLHandle: THandle; set_restore: set_func; remove_restore: remove_func; function begin_restore(var seqnum: int64; instr: widestring): DWord; function end_restore(seqnum: int64): DWord; function cancel_restore(seqnum: int64): integer; function error_report(inerr: integer): string; implementation uses sysutils, dialogs; function begin_restore(var seqnum: int64; instr: widestring): DWord; { starts a restore point } var r_point: restoreptinfo; smgr: statemgrstatus; fret: boolean; retval: integer; begin retval := 0; fillchar(r_point, sizeof(r_point), 0); fillchar(smgr, sizeof(smgr), 0); r_point.dwEventType := BEGIN_SYSTEM_CHANGE; r_point.dwRestorePtType := APPLICATION_INSTALL; move(instr[1], r_point.szDescription, max_desc); r_point.llSequenceNumber := 0; fret := set_restore(@r_point, @smgr); if fret = false then retval := smgr.nStatus; seqnum := smgr.llSequenceNumber; begin_restore := retval; end; function end_restore(seqnum: int64): DWord; { ends restore point } var r_point: restoreptinfo; smgr: statemgrstatus; fret: boolean; retval: integer; begin retval := 0; fillchar(r_point, sizeof(r_point), 0); fillchar(smgr, sizeof(smgr), 0); r_point.dwEventType := END_SYSTEM_CHANGE; r_point.llSequenceNumber := seqnum; fret := set_restore(@r_point, @smgr); if fret = false then retval := smgr.nStatus; end_restore := retval; end; function cancel_restore(seqnum: int64): integer; { cancels restore point in progress} var r_point: restoreptinfo; smgr: statemgrstatus; retval: integer; fret: boolean; begin retval := 0; r_point.dwEventType := END_SYSTEM_CHANGE; r_point.dwRestorePtType := CANCELLED_OPERATION; r_point.llSequenceNumber := seqnum; fret := set_restore(@r_point, @smgr); if fret = false then retval := smgr.nStatus; cancel_restore := retval; end; function error_report(inerr: integer): string; { error reporting, takes error, returns string } const SERROR_SUCCESS = 'Call Successful.'; SERROR_BAD_ENVIRONMENT = 'The function was called in safe mode.'; SERROR_DISK_FULL = 'System Restore is in Standby Mode because disk space is low.'; SERROR_FILE_EXISTS = 'Pending file rename operations exist.'; SERROR_INTERNAL_ERROR = 'An internal error occurred.'; SERROR_INVALID_DATA = 'The sequence number is invalid.'; SERROR_SERVICE_DISABLED = 'System Restore is disabled.'; SERROR_TIMEOUT = 'The call timed out.'; begin case inerr of ERROR_SUCCESS: error_report := SERROR_SUCCESS; ERROR_BAD_ENVIRONMENT: error_report := SERROR_BAD_ENVIRONMENT; ERROR_DISK_FULL: error_report := SERROR_DISK_FULL; ERROR_FILE_EXISTS: error_report := SERROR_FILE_EXISTS; ERROR_INTERNAL_ERROR: error_report := SERROR_INTERNAL_ERROR; ERROR_INVALID_DATA: error_report := SERROR_INVALID_DATA; ERROR_SERVICE_DISABLED: error_report := SERROR_SERVICE_DISABLED; ERROR_TIMEOUT: error_report := SERROR_TIMEOUT; else error_report := IntToStr(inerr); end; end; initialization { find library functions and enable them } DLLHandle := LoadLibraryW('SRCLIENT.DLL'); if DLLHandle <> 0 then begin @set_restore := GetProcAddress(DLLHandle, 'SRSetRestorePointW'); if @set_restore = nil then begin messagedlg('Did not find SRSetRestorePointW', mtWarning, [mbOK], 0); halt(1); end; @remove_restore := GetProcAddress(DLLHandle, 'SRRemoveRestorePoint'); if @remove_restore = nil then begin messagedlg('Did not find SRRemoveRestorePoint', mtWarning, [mbOK], 0); halt(1); end; end else begin messagedlg('System Restore Interface Not Present.', mtWarning, [mbOK], 0); halt(1); end; finalization { release library } FreeLibrary(DLLHandle); end. Sample code (use it in a form with two buttons and a label): unit srtool; interface uses Windows, Messages, SysUtils, Classes, Graphics, Controls, Forms, Dialogs, StdCtrls, sysrestore; type TForm1 = class(TForm) Label1: TLabel; Button1: TButton; Button2: TButton; procedure Button1Click(Sender: TObject); procedure Button2Click(Sender: TObject); private { Private declarations } public { Public declarations } end; var Form1: TForm1; implementation {$R *.DFM} procedure TForm1.Button1Click(Sender: TObject); { demonstration of making a system restore session } var seqnum: int64; retval: integer; testfile: TextFile; i: integer; inputstring: string; begin InputString:= InputBox('Input Box', 'Prompt', 'Test System Restore Session'); seqnum := 0; retval := begin_restore(seqnum, WideString(inputstring)); if retval = 0 then label1.caption := 'System Restore Entry Set: ' + IntToStr(trunc(seqnum)) else label1.caption := 'Error Str1: ' + error_report(retval); MessageDlg(label1.caption, mtInformation, [mbOK], 0); { do stuff here we want to back out } AssignFile(testfile, 'TEST.DLL'); rewrite(testfile); for i := 1 to 500000 do begin writeln(testfile, i); application.processmessages; end; closeFile(testfile); { end do stuff here we want to back out of } label1.caption := 'Finished.'; retval := end_restore(seqnum); if retval <> 0 then label1.caption := 'Error Str2: ' + error_report(retval); MessageDlg(label1.caption, mtInformation, [mbOK], 0); end; procedure TForm1.Button2Click(Sender: TObject); { clear system restore } var inresult: DWord; i: integer; seqnum: int64; topnum: integer; begin label1.caption := 'Please wait, cleaning system restore.'; application.processmessages; { get last sequence number } begin_restore(seqnum, 'Test'); cancel_restore(seqnum); topnum := trunc(seqnum) - 1; inresult := 0; i := topnum; while inresult = 0 do begin inresult := remove_restore(i); dec(i); end; label1.caption := 'Now Done.'; MessageDlg(label1.caption, mtInformation, [mbOK], 0); end; end. I can dig up the actual files and throw 'em into a ZIP if people need them, but I don't have them handy right now.
-
Get Key
Glenn9999 replied to gunsmokingman's topic in Programming (C++, Delphi, VB/VBS, CMD/batch, etc.)
I meant that the other way around, not many of us around to answer such questions for others. True. Why is this? Really it's because 95% of this sub-forum on MSFN is all batch and scripting (and nothing wrong with that, it's one of the top topics in one of the hardcore programming forums I referred to below). There are people that know these things on here and are getting a workout from what I can tell, but it's kind of hard to want to participate if nothing comes up that's in your field of expertise or interest. That's my category, to be honest. There's programming things I could post and things I could help on, but they never seem to come up in here, and I'm really about 90% sure from the posts here that enough would be interested to count on one hand what I would post. I could always roll the dice and post a couple of things that are semi-relevant to the other topics on this board, though... Now, my interest in this site and participating in this forum is more of a software and hardware nature. I get value from those things, and I get value in the technicals of the OS for what programming projects I do do. I also have posted a couple of my semi-completed projects on here, to mild notice (and that's fine as long as they're useful to someone - they seemed to be, which makes it worthwhile to me to post them, and I have no regrets in posting them). Now, I participate in a 2 or 3 other forums that are more hardcore towards "actual programming" (as CoffeeFiend puts it), and the large preponderance of people are people that do programming for a living (I'd say 99%). For a couple of the topics, it seems I'm the hobbyist sitting among everyone else who is making a buck doing what is involved in the forums (and some accomplished enough that you could call them luminaries in their chosen competency). And many of them participate, ask questions, answer them occasionally, and yes even profess then and again the usefulness of such forums to them. My thoughts on these things? Usually, people tend to participate and flock to things that have others who are interested in the topic as well. It's a initial gauge I know most put up when they first see something - no posts on what I'm interested in? Maybe I should move on. Posts on what I'm interested in? Let me stay awhile. Of course, any site can't be everything to everyone. A forum is what the aggregate of its posting members make of it. Now, let me go through my source and see what I could post here... -
I think it may have hastened the hard drive's departure (the one I referred to in the original post). I used the hard drive diag from the manufacturer to test sectors for readability amongst other things and it registered there was a problem. Anyhow with a NTFS partition, what is a suitable tool to do this job (assuming good drive but corrupted data structure) that is effective and won't hose the drive? Or is it just hope and pray with such things? At least with a FAT32 partition, you could load a physical boot disk and run SCANDISK or NDD there, but can't exactly do that with NTFS...
-
I had another experience regarding poor support of disk checking in Windows XP. Anyway, once upon a time I had a hard drive fail and the disk check option didn't show anything while I was losing files right and left with errors abound, while the disk check would merrily go on with nothing to report. Now, I had a floppy disk with unreadable files and the disk check in XP did nothing. Thankfully, I had my brand new Windows ME VM, so I booted there and ran the Scandisk there and it fixed it right up (the LFNs got trashed on three of the files). So do most people do this kind of thing (or similar, like a DOS boot disk?) when it comes to disk checks, or is there something I'm missing that's wrong with XP (a patch or something) that would make this function actually work properly? I would think these days that a properly working chkdsk would be an expectation. Or not?
-
Ah yes...I forgot about that one. My first computer was a Packard Bell. 486SX/25. That one was so bad that I guess I blocked it out. Mainly due to tech support issues over its proprietary nature. A screaming match over getting the modem riser out of it so I could run a replacement modem - basically they wanted to charge me $150 after I took a 80 mile trip just to install a jumper that they wouldn't send (finally they sent it). Final straw was the board failing and them wanting $800 to replace it. So I ended up taking about half that and salvaging some usable parts out of the Packard Bell and assembling my first custom computer (with jumpered I/O board - be thankful for what you have today if you don't know what I refer to!) and never looked back. Only regrets, really, were some bad parts because of limited funds. Unfortunately, I didn't get to have any Office Space moments with the Packard Bell, but that definitely was an epic fail.
-
Either you haven't linked the physical CD-ROM into the VM (do that and reboot the VM), or you need a boot floppy (i.e. the disk is an upgrade), and you need to link the floppy drive to the VM when you use it and reboot the VM. Hope that helps.
-
It has turned into a real security concern. But Microsoft only patches what they want to patch. As my understanding goes, this is a real Vista security patch, yet it hasn't shown up on XP - wonder why? (and yes I know the answer) See http://www.us-cert.gov/cas/techalerts/TA09-020A.html http://www.msfn.org/board/index.php?showtopic=129051 http://www.msfn.org/board/index.php?showtopic=128880
-
http://sourceforge.net/projects/pdfcreator/ You don't say what OS, but if you're on Windows this will do it.
-
I just ran across this page, posted January 21, 2009 and thought it might be interesting reading. http://www.us-cert.gov/cas/techalerts/TA09-020A.html Overview Disabling AutoRun on Microsoft Windows systems can help prevent the spread of malicious code. However, Microsoft's guidelines for disabling AutoRun are not fully effective, which could be considered a vulnerability.
-
I thought of this one...I'll say the first thing that comes to mind is the Vanilla Ice CD from back in 1990.
-
Some more sharing of DOS stuff. But apps this time (I posted most of my driver collection that was out of the ordinary). Though I noticed when I was setting up a DOS VM that I have no CPU idling drivers. So I'm sure there are more categories that have been missed. Anyway to the apps: MP3 players? Here's one that has most of the bells and whistles that you can get out of DOS, as long as you use the right command-line parms: http://www.damp-mp3.co.uk/ There's also a link to a Media manager on the site, as well as some ID3 editing tools. Another one. Need a CD-burning software for DOS? http://www.freeweb.hu/doscdroast/ http://www.goldenhawk.com/dos.htm And a third, though I hesitate to post it for the warez rules (I'm not entirely sure of its status). Did you know that version 1.0 of the Acrobat Reader (you know PDFs?) was written as a DOS app?
-
2.10, though recently upgraded to 2.12. Can't be much more specific than what I spoke of. But here you go. Case #1 was fixed with the upgrade to 2.12, though here are three more. Locked up. http://bnfbua.bay.livefilestore.com/y1pVm7...EQ/VBOXSS02.JPG http://bnfbua.bay.livefilestore.com/y1pIoz...yA/VBOXSS03.JPG http://bnfbua.bay.livefilestore.com/y1pxLM...xQ/VBOXSS04.JPG DOS, as can be seen from the screenshots. Windows ME (again screenshots). Though it never got loaded except off the vanilla bootdisk. Slow as molasses on a 0F day, but that's a different problem. JO.SYS. See the first screenshot.
-
if the cd or the iso is bootable, I can't see why it would choke. Maybe your memory repartition is such that your host OS chokes and VirtualBox freezes. Well let's see if I remember the littany of problems: 1) One boot disk only came up with a line of garbage. 2) Another boot disk hung after loading JO.SYS. 3) Yet another standard (yes the one that it will make if you tell it to on install) Windows ME system disk hung on one of the Adaptec ASPI drivers. All three work fine on the machine if booted straight from them and not from the VM and all three have no bad sectors or corrupt files.
-
OK I figured this one out, finally. I ended up making a very vanilla boot disk (bare minimum of OS files & CD-ROM drivers) and it worked. Evidently, VirtualBox chokes on anything non-vanilla. I tried a good number of combinations of things (basically all my OS boot disks - if you can't load an OS onto the VM, you can't get much farther). Got an old copy of Windows loading now, so it seems like things are under control. Thanks for the response.
-
The only thing that keeps one from using Virtual PC on home OSes is the EULA (I've seen it working on a copy of XP Home - Vista might be different of course).
-
That helps. Thanks. It shouldn't be too much trouble from here to set up a batch file to run this against all the plugins.
-
I have tried VirtualBox on several occasions, but the problem I have is that I see nothing but a line of garbage come up when I try to set up a virtual hard drive with any of my boot disks. Any suggestions on how to solve? Thanks.
-
I've been searching (and probably haven't used the right words), and haven't found this answer so I'm asking. Is there a way to get an offline collection of plugins and have them installed either along with Firefox or separately so when Firefox loads it will go ahead and self-update the plugins or just fire up with the plugins in operation?
-
turbo c dos based compiler.
Glenn9999 replied to ey_pril's topic in Programming (C++, Delphi, VB/VBS, CMD/batch, etc.)
http://dn.codegear.com/article/20841 Though I'm surprised that anyone would use such an ancient option when something (many somethings) both better and free is out there. -
90% just now. Though I've done better. Edit: Just now. 94% And now, 100% (it's an image): http://bnfbua.bay.livefilestore.com/y1puQY...%20freakout.jpg
-
Are All or Some of These NET Framework Updates Necessary?
Glenn9999 replied to HoppaLong's topic in Windows XP
The three updates are listed on the page. When .NET 3.5 is installed, all three things are installed. So if you are looking for updates, having the first .NET 3.5 (not service pack 1) installed, then download all 3. If you downloaded the full .NET 3.5 package and it's dated at the same time as the other patches, then you should be fine and not need to update anything. -
The link doesn't expire.
-
http://www.computerworld.com/action/articl...ticleId=9123118 http://www.computerworld.com/action/articl...tsrc=hm_ts_head http://www.msnbc.msn.com/id/28258894 REDMOND, Wash. - Microsoft Corp. is taking the unusual step of issuing an emergency fix for a security hole in its Internet Explorer software that has exposed millions of users to having their computers taken over by hackers. Microsoft said it has seen attacks targeting the flaw only in Internet Explorer 7, the most widely used version, but has cautioned that all other current editions of the browser are vulnerable. http://www.microsoft.com/technet/security/...n/ms08-dec.mspx It effects everything Internet Explorer. Probably related to this: http://www.microsoft.com/technet/security/...ory/961051.mspx