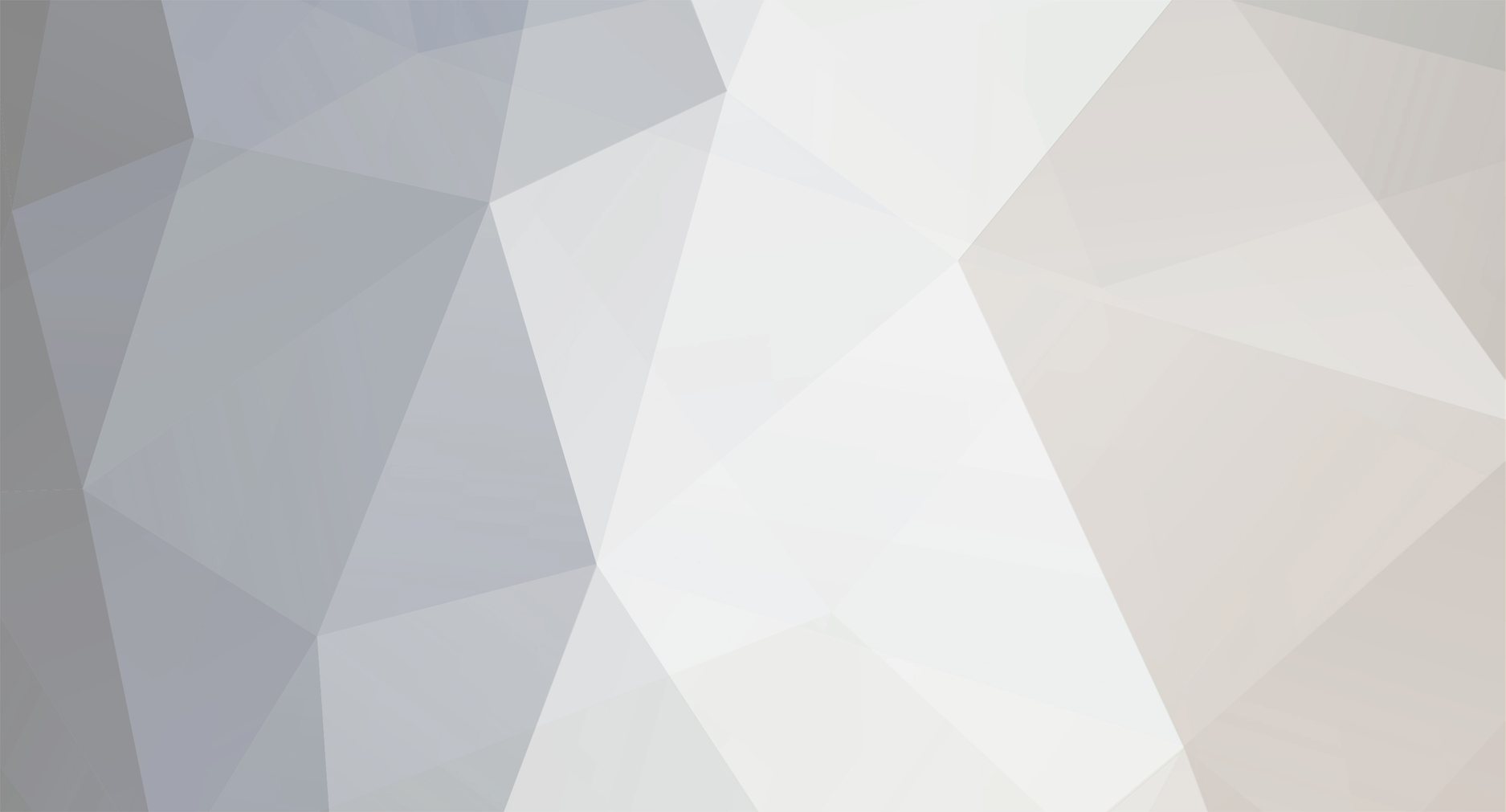
zeusabj
Content Type
Profiles
Forums
Events
Posts posted by zeusabj
-
-
Now see, that's why it says "Wise Owl" under your avatar. Seriously, thank you *so* very much for taking the time to write up that reply. That's the exact kind of constructive criticism I have been looking for. I've only been writing batch scripts for about a year now and I'm embarrassed to say there's still a lot I don't understand. Also I'm located in a small town and there aren't many other technical people around, so I rely on the Internet a lot to bounce questions off other technical individuals. Would you believe it took me almost two whole days to come up with that code on my own? If I'd have just had someone local to ASK in the first place, then I thought of MSFN. You guys totally came through for me.
Thank you so much!
0 -
This was my final solution, and it seems to work well:
@ECHO OFF
:: Prepare the Command Processor
SETLOCAL ENABLEEXTENSIONS
SETLOCAL ENABLEDELAYEDEXPANSION
:: Defaults (directory paths must be in quotes):
::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
SET _source="C:\temp\copy 1"
SET _destination="C:\temp\dest 1" "C:\temp\dest 2" "C:\temp\dest 3" "C:\temp\dest 4" "C:\temp\dest 5"
SET _options=/MIR /XJ /NJH /NDL /NJS /NS /NC /R:1 /W:10
:: Customize What Gets Copied
::SET _filelist=
::SET _excludedfiles=/XF
::SET _excludedirs=/XD
:: Confirmation:
::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
ECHO -------------------------------------------------------------------------------
ECHO Robocopy Backup Script:
ECHO -------------------------------------------------------------------------------
ECHO.
ECHO Backup Source:
ECHO --------------
ECHO %_source%
ECHO.
ECHO Backup Destination(s):
ECHO ----------------------
FOR %%G IN (%_destination%) DO ECHO %%G
ECHO.
ECHO Press CTRL+C at any time to abort the backup job.
ECHO -------------------------------------------------------------------------------
SET /P continue=Proceed? (Y/N):
IF /I %continue%==N GOTO _END
:: Run Backup Job
::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
CLS
ECHO.
ECHO Updating Destination(s):
ECHO ------------------------
FOR %%G IN (%_destination%) DO ECHO. & ECHO -Updating %%G & ROBOCOPY.EXE %_source% %%G %_excludedfiles% %_excludedirs% %_options%
GOTO _END
:: End:
::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
:_END
ECHO.
ECHO Backup job complete, press any key to exit...
PAUSE > NULWhat do you guys think? See any potential "gotchas" in my code that could cause it to mess up? I guess I'm worried that it seems a bit "rigged" what with all the "&"'s in my FOR loop. Any suggestions to improve it?
0 -
I hope this suits your purpose!
@echo off
:: Defaults:
:::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
:: Set variables
set _sourcedir=C:\temp\copy1
set _options=/MIR /XJ /NJH /NDL /NJS /NS /NC /R:1 /W:10
set _destlist=H:\Backups "F:\Backup Drive" E:\Backup_2
:: Loop through list of destination directories
for %%# in (%_destlist%) do call :_continue %%~#
:: Exit:
:::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
:_end
echo=
echo=Backup job complete, press any key to exit...
pause>nul
goto :eof
:: Confirmation:
:::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
:_continue
set _destdir=%*
cls
echo=-------------------------------------------------------------------------^
------
echo=Backup Script:
echo=-------------------------------------------------------------------------^
------
echo=
echo=Backup Source folder(s):
echo ------------------------
echo=[%_sourcedir%]
echo=
echo Backup Destination folder(s):
echo=-----------------------------
echo=[%_destdir%]
echo=
echo=Press CTRL+C at any time to stop the transfer.
echo=
set/p "continue=Proceed? [Y/N]: "
if /i %continue%' neq y' goto :eof
:: Run Backup Job
:::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
cls
echo=
echo=Updating [%_destdir%]:
robocopy "%_sourcedir%" "%_destdir%" %_options%
pauseAwesome, I never realized it could be as simple as putting spaces between the times in my variable declaration. I'll try that and post my final code here for you guys to look at.
0 -
I won't bore you with the details, just know that this has to be a BATCH SCRIPT (I can't do this using another language or program and achieve the desired affect).
Here's my current script:
@echo off
:: Defaults:
::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
:: Backup 01
set _sourcedir01=C:\temp\copy1
set _destdir01=H:\Backups\
set _options01=/MIR /XJ /NJH /NDL /NJS /NS /NC /R:1 /W:10
:: Confirmation:
::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
echo -------------------------------------------------------------------------------
echo Backup Script:
echo -------------------------------------------------------------------------------
echo.
echo Backup Source folder(s):
echo ------------------------
echo [%_sourcedir01%]
echo.
echo Backup Destination folder(s):
echo -----------------------------
echo [%_destdir01%]
echo.
echo Press CTRL+C at any time to stop the transfer.
echo.
set /p continue=Proceed? (Y/N):
if /i %continue%==N goto _end
:: Run Backup Job
::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
cls
echo.
echo Updating [%_destdir01%]:
robocopy "%_sourcedir01%" "%_destdir01%" %_options01%
goto _end
:: Exit:
::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
:_end
echo.
echo Backup job complete, press any key to exit...
pause > nulSo simple and it works right? Here's my problem. I need to somehow tweak this so that I can copy to multiple destinations. Now I know I could just create a new variable for each destination and then run each variable through this own robocopy command, but I'm thinking there has to be an easier way to do that and reuse code. I understand batch scripts can't do arrays, but I'm thinking something similar is what I need. Here's some pseudocode that (I hope) illustrates my goal:
@echo off
:: Defaults:
::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
:: Backup 01
set _sourcedir=C:\temp\copy1
set _destdir="'H:\Backups\', 'F:\Backup Drive\', 'E:\Backup_2'"
set _options=/MIR /XJ /NJH /NDL /NJS /NS /NC /R:1 /W:10
:: Run Backup Job
::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
loop through values in %_destdir%
robocopy "%_sourcedir%" "%_destdir%" %_options%
end loopAs you can see from the above pseudocode all I really want to do is somehow set up a series of directory paths and pass each one through to my %_desdir% variable in sequence. My intended solution also needs to support spaces in directory paths. Does anyone know how to do this?
0 -
Thanks for sharing this. Always love to know what items other SysAdmins are setting as default in their Windows installs. I incorporated a few of your tweaks into my own post-install script.
0 -
If anyone is interested I found an excellent solution for this dilemma here:
http://social.technet.microsoft.com/Forums/en/mdt/thread/54f71ff7-81b6-4dff-9fb1-845a2efb1026
Aaaaand here is the script I wrote to do the work for me (with help from that post):
@echo off
:: Set script execution directory
set _thisdir=%~dp0
:: Take ownership of original
TakeOwn.exe /F %SystemRoot%\Web\Wallpaper\Windows\img0.jpg
:: Replaces acls with default inherited acls
icacls %SystemRoot%\Web\Wallpaper\Windows\img0.jpg /reset
:: Rename original
RENAME %SystemRoot%\Web\Wallpaper\Windows\img0.jpg imgX.jpg
:: Run copy operation
XCOPY "%_thisdir%Wallpaper\*.*" "%SystemRoot%\Web\Wallpaper\Windows" /D /E /C /R /I /K /YHope this makes it easier on the next person!
0 -
mount and write, may need to take ownershipDo you have a workaround for the "TrustedInstaller" permissions issue, or do you just mount an image and overwrite it in there?
Yeah figured, ah well, at least I have a solution now, thanks guys!
0 -
C:\Windows\Web\Wallpaper\Windows\img0.jpg
yes I do that also and replace it everywhere on the drive. You'll find it in several more places
4 places on a 64 bit and then you can also replace
AppData\Roaming\Microsoft\Windows\Themes\TranscodedWallpaper.jpg with same.
Do you have a workaround for the "TrustedInstaller" permissions issue, or do you just mount an image and overwrite it in there?
0 -
Well, I knew I wasn't crazy, check this out:
http://social.msdn.microsoft.com/Forums/en/windowsuidevelopment/thread/1703c3f4-a2ea-4c4a-a73f-a93e6d51b2bb
So looks like my only solution is to overwrite this wallpaper file:
Which seems like a rather sloppy approach, but hey if it works....
0 -
Change it to REG ADD "HKU\Hive....... Which you would have known had you read the link I originally posted.
REM Load the default profile hive
REG LOAD HKU\Hive C:\Users\Default\NTUSER.DAT
REM Configure the default user profile
REG ADD "HKU\Hive\Control Panel\Desktop" /v WallPaper /t REG_SZ /d "C:\Windows\Web\Wallpaper\Nature\img6.jpg" /f
REM Unload the default profile hive
REG UNLOAD HKU\HiveI'm sorry Mr. Jinje! I did read your link! That was a typo! I pasted a code snippet from an earlier attempt! I did find and correct that error *BEFORE* I posted that response. So the script I ran actually did use 'REG ADD "HKU\Hive.......' and it still did not work. I have edited my above post to remove the typo. Just to make sure it *would* work I decided to make another script and apply the registry key for the user currently logged in. Here's my script:
:: Change Wallpaper
REG ADD "HKCU\Control Panel\Desktop" /v Wallpaper /t REG_SZ /d "C:\Windows\Web\Wallpaper\Nature\img6.jpg" /f
:: Refresh User Settings:
%SystemRoot%\System32\RUNDLL32.EXE user32.dllThis did change the registry key for the current user and (after a reboot) the custom wallpaper *was* applied! Now if I could just figure out why it is failing when I edit "C:\Users\Default\NTUSER.DAT". I did notice when I checked the registry entry for the first time after I logged into the new user account (that was supposed to contain my custom settings) I found that it was overwritten by the default wallpaper location which is "C:\Users\<Username>\Appdata\Roaming\Microsoft\Windows\Themes\TranscodedWallpaper.jpg". So in short, if I modify the registry entry manually, it gets reflected properly. This suggests that Windows 7 is "doing something" to change my settings when a new user logs in for the first time.
Thoughts?
0 -
So I changed my code to this:
REM Load the default profile hive
REG LOAD HKU\Hive C:\Users\Default\NTUSER.DAT
REM Configure the default user profile
REG ADD "HKU\Hive\Control Panel\Desktop" /v WallPaper /t REG_SZ /d "C:\Windows\Web\Wallpaper\Nature\img6.jpg" /f
REM Unload the default profile hive
REG UNLOAD HKU\HiveAll the commands run successfully. So I reboot, create a new account, login as the user, and still no custom wallpaper. I'm just at a total loss! What in the world am I doing wrong here? Has anyone ever actually gotten this default registry hive thing to work? Can someone maybe give me a code snippet that they have confirmed to work so I can at least know it does do something?
Please tell me someone knows the answer here.
0 -
I am running this from the administrator profile, rebooting the computer. Creating a new user account from scratch and then logging in as that user to test. Shouldn't that work?
Yep, that should work (but only on a per computer basis), I don't know about the path in your sample, look at the sample from post #6 in the thread I linked.
No such thing a "Default User" anymore, you are editing the wrong path entirely. In windows 7 Default User is now "Default". Just change your path to C:\Users\Default\ntuser.dat, instead of %USERPROFILE%\..\ Maybe that is the whole problem.
reg load "hku\zzz" "C:\Users\Default\NTUSER.DAT"
REG LOAD "HKU\Default" "%USERPROFILE%\..\Default User\NTUSER.DAT"
I was under the impression that using "Default User" would still work because there is a junction point in place for that (do a "cd C:\Users\Default User\" at a CMD prompt and you'll see what I mean. Plus the above site's code also said to use default user, so i just assumed it would work. Maybe the junction point is jacking it up. I'll try your suggestion.
0 -
He is trying to modify NTUSER.DAT which you must make sure you have the image mounted and modify the one within the install.wim. I just see that he needed to change the Load Hive name maybe cause it would conflict.
MrJinje
You can add all that stuff directly as he is trying to do.
So hold the phone, this can't be done within windows? I have to create the image first, then mount it, then run these commands? I have been doing my deployment to a VM and logging in as Administrator, then running this *within* the Windows 7 install I intend to capture. Could that me my problem?
0 -
Here is an older thread on the subject, you should modify the hive from setupcomplete.cmd so that it gets pushed into the default profile before the first user profile is created. If you wait until the first login, the settings will not apply to that first profile, but only to profiles created after the changes are made.
I am running this from the administrator profile, rebooting the computer. Creating a new user account from scratch and then logging in as that user to test. Shouldn't that work?
0 -
try changing HKU\Default to different like HKU\MyTest
I see a default value in root of HKU
Okay I'll give that a try and report back.
0 -
Hey guys,
I really hope someone can help me here because I'm at a loss here. I've been tasked with creating a Windows 7 image for deployment at my company. When I built our Windows XP images a few years ago it was pretty easy to set system-wide defaults. All I would do was create a dummy account, log in to that acount, make my customizations (default company wallpaper, disable "highlight new programs", etc) and then just overwrite the "Default" profile folder with the profile folder of the dummy account. When I got ready to start deploying Windows 7 I did some research and discovered that method was no longer supported. After looking into the methods that were supported I found this page:
http://windowsteamblog.com/blogs/springboard/archive/2009/10/30/configuring-default-user-settings-full-update-for-windows-7.aspx
I looked at what I wanted to accomplish and decided to go with what that site refers to as "option C", or "Targeted changes to the Default User Registry hive and profile folders". I took the code snippet they provided on their site and tried to adapt it to my purposes, but it does not appear to be working. Here's a sample of my code that is *supposed* to set a default wallpaper for every new user that logs in:
:: Load the default user hive, set Wallpaper value, unload Default User Hive when doneREG LOAD "HKU\Default" "%USERPROFILE%\..\Default User\NTUSER.DAT"REG ADD "HKU\Default\Control Panel\Desktop" /v WallPaper /t REG_SZ /d "C:\Windows\Web\Wallpaper\CompanyName\CompanyImage" /fREG UNLOAD HKU\Default
For the life of me I can't figure out why this is not working. Can anyone tell me what I'm doing wrong here?
0 -
Ashinator...
What can I say? *THANK YOU* you just have no idea how long I have been trolling the web for a *SIMPLE* step-by-step guide WITH SCREENSHOTS that just spells out how to create hardware independent sysprepped WIM images. I keep finding bits and pieces of the process but nothing that spells out the ENTIRE process for you completely from cradle to grave. You have no idea how much easier this will make things for me at work. I simply can't thank you enough for this.
YOU ROCK!
0 -
You could very well use a specific set of tasks within you sequence for each model. You would put in logic that uses WMI to determine the model of the hardware and then run particular tasks based on that. A bit complicated, but doable.
I would focus on the hardware that does not have the drivers installed correctly for. It could be that the hardware does not have a PNP driver but requires an installation of the software.
The process is working, it's just not finding matching drivers for the hardware that it is skipping.
Sorry I have not responded had a number of work and family related items that came up ad just slammed me. Thanks for your help MMarable. I guess I'm just going to have to sit down and figure it out through trial and error. never done any scripting like that beofre. Sure wish there was some sort of tutorial that could help me. Oh well. If anyone finds any good MDT-related guides that describe my problem and detail some solutions, please post them!
0 -
Are you getting any device drivers installed? I tried a quick MDT build using XP-SP2 and it worked for the most part. ZTIDrivers.wsf inventories the PCI IDs of the hardware it finds on the box and the compares that to the driver store in MDT. When it finds a match it copies it to the C:\Drivers (hidden) and updates the DevicePath in the Registry. PNP does the rest.
What could trip it up is if the "Inject Drivers" task is disabled in the Task Sequence, or the drivers you have do not match up with the hardware (ie Vista drivers for XP machine).
Do you have any drivers installing successfully?
Do you have a hidden "Drivers" folder off the root of your C: drive?
If so, what is in it?
Are the paths found in C:\Drivers listed in the DevicePath key in HKLM\Software\Microsoft\Windows\CurrentVersion?
Okay you offered some interesting tips. I'm still really new to all of this and you made me aware of quite a few things. Yes, the hidden drivers folder is there, and it seems some of the drivers *did* install while some did not. Mainly mass Storage controllers, NIC and video drivers. When I look in that folder it appears to have copied over five driver files and those appear to have installed properly. Is there some other step I should take here? I guess I'm just assuming the LiteTouch ISO is properly detecting my hardware and installing the appropriate driver. Is there some way to "tell it" what to do? Maybe create a task sequence for each model? If so how do I tell it where to find the drivers?
I know I'm asking a lot of questions here, but I've read a lot of the documentation and none of it seems to cover my specific requirements (running the entire thing from a single DVD). Surely I can't be the only person trying to do this. Are there any sort of step-by-step guides or how to's out there with screenshots that can help me?
0 -
Hey guys,
I'm totaly hung up on this issue and I'm really hoping somsone here can help. I am using the MDT 2008 to standardize OS deployment for my company. Our goal is to have a single DVD that can be used to load Windows XP SP3 (Applications, Drivers and all) onto a new PC or be used to refurbish existing models deployed in the field as they come in for maintenance/repair. I have been able to use the Media Deplyment point to successfully create and test several ISO's. So far they install our company applications without any issue, its the DRIVERS that are killing me! I got all the drivers for every model we have extracted and imported into the Out-Of-Box drivers, but for some reason they don't install when I run the ISO. I can launch device manager after Windows is installed, choose the option to update drivers, browse to the DVD, find the appropriate driver and it installs perfectly, but I was under the impression the MDT would install this automatically (if you had them proplery set up in Out-Of-Box Drivers). What am I doing wrong here? Any ideas?
I've searched the forum and have not found an answer to this, maybe I was not uing the right search terms. Can anyone help?
0 -
To answer the "why" questions, I have several technicians that work at my company that were issued these USB Western Digital passports to use on the job. Several of them prefer these devices to USB flash drives due to their high storage capacity. I recently created a Windows PE-based custom technician recovery install that I have been setting for the technicians that have Flash drives. Because of the Econo-apocalypse our company has put a freeze on purchases and the guys with the USB drives are asking me to come up with a solution for them. Due to the 32GB limitation of FAT32 in DISKPART I need to come up with some sport of NTFS-based bootable solution to allow them to use the entire capacity of their drives without having to make two partitions. I've stepped through several guides but so far I get an error every time I boot from the drive. Although the errors have varied some my current error is as follows:
"A disk read error occurred Press CTRL-ALT-DEL to restart"
I hope that explains things better.
It can be done. There is a guide in the WAIK..I have a 80 gb notebook drive in a USB2 ext enclosure.. I use it for storage and emergencies.. Boots right up to PE if I tell the bios to do so. Othewise, PE sits along side (single NTFS partition BTW) my other files (backups mostly) just waiting for the day I need to boot to it.
Bilemke, so the AIK guide worked for you. I call myself stepping through this guide, but maybe I made a mistake. I'll try it again...
Also thanks so much for the responses guys, I appreciate the help!
0 -
Hey guys,
I'm trying to get Windows PE to boot from a 300 GB 2.5" Western Digital Passport external USB drive. To get around the FAT32 partition limitation of 32GB in DISKPART I've opted to format to NTFS. I've found several guides for making an NTFS formatted drive bootable, but none of them seem to be working for me. I just get an error every time I specify the external drive in the boot menu of my target machine. I was just wondering if anyone here has tried and succeeded in making a high capacity 2.5 external USB drive boot into Windows PE? If so could you please describe the process or link to the guide you used. Getting this figured out would be a tremendous help to me.
Thanks in advance!
0 -
IT WORKED Tripredacus!!! PERFECT! This was exactly what I was looking for, thanks! On a related note I have not had a chance to test it on an APPLY job. Will the same key combination abort ImageX when I am applying an image? This would be useful if I make a mistake and apply the wrong image or something...
0 -
I'm pretty sure you can Ctrl+C it... You'll lose your partially created WIM tho after you close it.
Something like this was what I was looking for. CTRL+C huh? I'll try that and report back...
0
Need help with a batch script using robocopy...
in Programming (C++, Delphi, VB/VBS, CMD/batch, etc.)
Posted · Edited by zeusabj
Hey Yzöwl, another dumb question. Why did you use "=" after each "echo"? Is there a reason for that or is it just your preferred programming style? Also I've seen other batch scripts that use "goto :eof". Obviously that is used to exit the batch script but you never see the actual ":eof" function anywhere in the script. Is it just implied or could I name it anything (like "goto :nothing"); leave ":nothing" out of my script and have it work the same way?